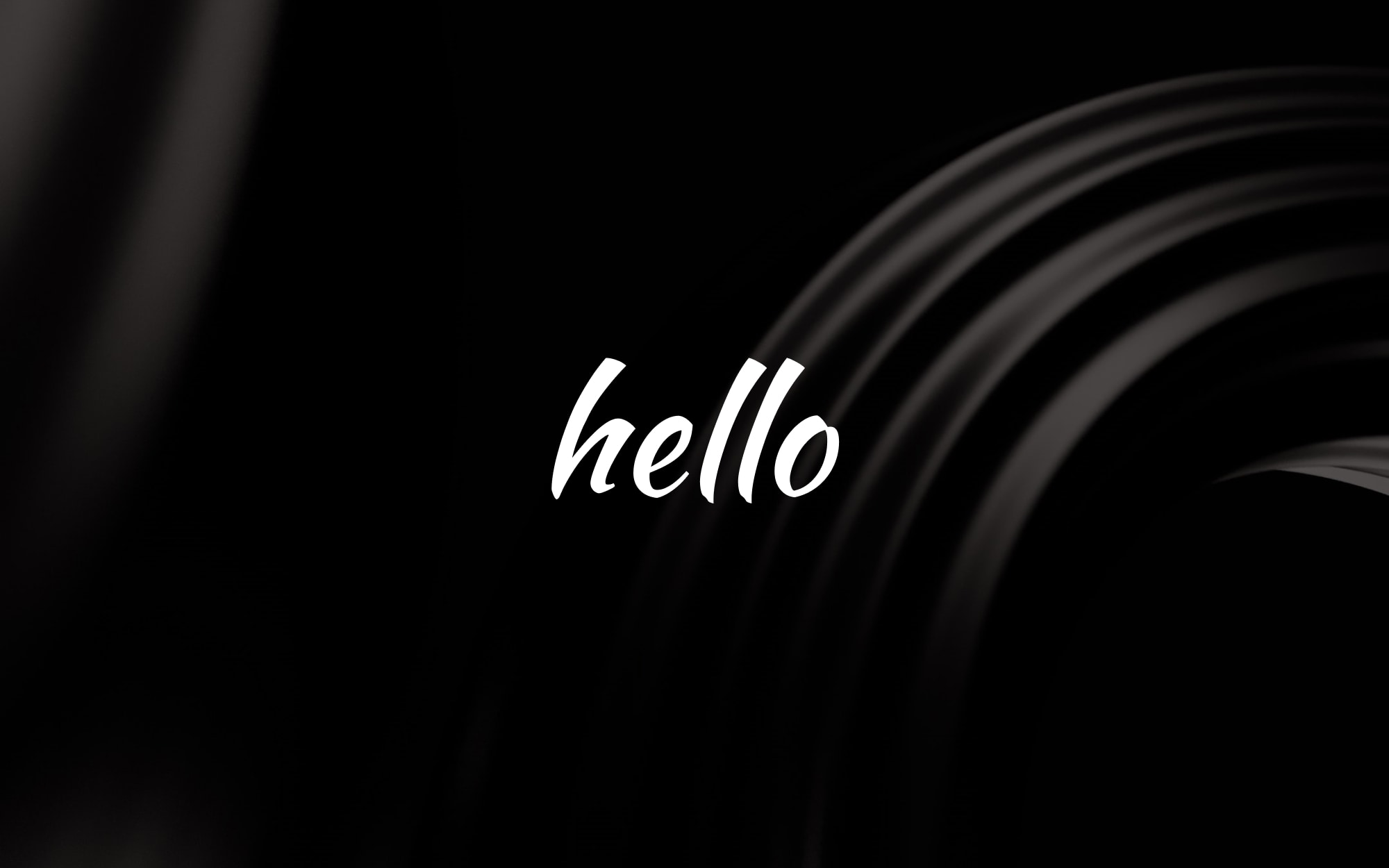
Coming soon
This is Sunset Raven, a brand new site by David James that's just getting started. Things will be up and running here shortly, but you can subscribe in the meantime if you'd like to stay up to date and receive emails when new content is published!